Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.

Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications You must be signed in to change notification settings
4_More_Assignment_Numbers_Strings.md
Latest commit, file metadata and controls, more about assignment, numbers and strings.
In lesson 2, we introduced the basics of working with numbers, expressions and variables: how to express numbers as literals, assign them to variables, and create numeric expressions using various operators. And in lesson 3 we got introduced to strings. In this lesson, we'll go into more detail on each of those topics.
Multiple assignment
- Using compound assignment operators
Range and precision of int and float
Different formats for numeric literals, bitwise operations on integers, strings as unicode character sequences, converting between number and string types, what's next.
We've already seen the familiar assignment operator, = , for assigning values to variables.
Something that's very handy in Python is that you can assign values to multiple variables in a single statement.
You simply list two or more variables on the left, separated by commas, and then the same number of values on the right, also separated by commas. There must be the same number of variables on the left as there are values on the right, otherwise you'll get an error.
The examples above have literals as values, but you can also use expressions or functions.
Using compound assigment operators
Like several other languages, Python supports compound (or in-place ) assigment operators that evaluate an expression and assign the result. For example:
In the second statement, += is used to combine two steps into one. First, the expression x + 5 is evaluated; then, the result is assigned back to x . It's convenient shorthand for x = x + 5 .
The other numeric operators also have corresponding compount assignment operators. Follow along this sequence of statements:
Note: some languages support =+ and =- as well as += and -= , with slightly different effects in looping conditions. In Python, =+ and =- are meaningul, but they're not compound assignment operators! They're simply the assignment operator = with the following + and - interpreted as prefixes to the following number. For example, >>> x =- 5 means exactly the same thing as >>> x = -5
With strings, += can be used as a compound concatenation/assignment operator.
Similarly, *= can be used as a compound repeat-concatenation/assignment operator; the following operand is an integer.
In Python, int s are not limited to a particular range or machine representation. For example:
The float type, however, however, has limited precision. The actual limitation will depend on the specific Python interpreter you're using and the architecture of the machine you're running on. You can enter a float literal with any number of decimal places, but the value will be constrained by the precision limitation of your setup.
Because of the precision limitation, rounding errors will occur, especially with certain numeric operations. We already saw some examples of this in lesson2.
There's a way you can get details about the supported precision of float on your system, but it involves using certain features we haven't explored yet (importing a module, sys , that's included with your Python installation but not loaded by default). If you want to find out more, see the Python documentation for sys.float_info . Here's an example of what the detailed info looks like:
To get an idea of how this compares to floating value types in other languages, this is comparable to the range for double in the Microsoft C++ compiler .
The float type in Python supports values for positive and negative infinity. These are obtained by passing certain strings to the float() function:
Either the short or long strings, 'inf' or 'infinity', can be used. Also, case doesn't matter.
Or course, most numeric calculations involving infinity result in infinity.
There are a few exceptions, but they involve another special float value, nan , meaning not a (well-defined) number . So, for example:
You can obtain the nan value directly as follows:
Note: nan is not the same as 0, null or None .
Because int s aren't bounded, these concepts don't apply. (Happy counting galaxies, Buzz Lightyear! When you're done, come join us at Milliways.)
So far, we've only dealt with numeric literals using decimal representations. But integer literals can also be expressed in hexadecimal, binary or octal. (Results in interactive mode will be shown in decimal, however.)
For hex, use the "0x" prefix followed by any number of digits; digits A through F can be entered in upper or lower case:
To write literals in binary, use the "0b" prefix. For octal, use the "0o" prefix.
Expressions using numeric operators can take literal operands expressed in any of these forms:
If you want to see integer values exprssed as hex, binary or octal strings, you can use the built-in hex() , bin() or oct() functions.
Note that these functions return strings, not integers!
In the previous section, we saw that ```int`` values are unbounded, so it's possible to work with some really long number literals. (And if you need to write numbers as binary, those can quickly get long as well.) In daily life, we use grouping delimiters to make it clearer what the value is. For instance
4,294,967,296
is much easier to read than
When writing long number literals in Python, an infix "_" can be used as a grouping delimiter.
Note that "_" must be an infix and can't occur at the start or end of an integer literal; that would result in an error. The Python interpreter will ignore any infixed "_", so any size grouping can be used. Be sure to stick to conventional groupings so that you don't get confused later:
For decimal digits, stick to groupings of three. (Some cultures use other sized groupings, but three is universally familiar.), For hex, use groupings of four or eight; and use eight for binary.
We've been talking about int literals. What about floats ? Hex, binary or octal representations are not supported for float s. You can use the "_" grouping delimiter, however.
Up to now, we've seen examples of floats represented normal, decimal representation. There is another representation that's also used for float literals, the exponent form:
The number after "e" is an exponent applied to a base of 10, and that is multiplied times the number before "e". For very large float values, results in interactive mode will use the exponent representation with a single-digit mantissa.
In addition to arithmetic operators seen earlier, Python has a typical set of bitwise operators.
There are also corresponding compound assignment operators for all but the bitwise complement.
Note that bitwise operations are only meaningful and supported for integers ( int ). Also, for shift operations, the second operand cannot be negative.
As in other languages, bitwise or , and or exclusive or set individual bits in a result value by comparing the corresponding bits in two operands:
- or: 1 if either operand bit is 1, else 0
- and: 1 if both operand bits are 1, else 0
- exclusive or: 1 if operand bits are different, else 0
Right shift causes binary digits to be shifted right by a specified number of digits. For example:
Right shift is equivalent to floor division by powers of 2. As existing digits shift right, 0 digits are inserted on the left; least-significant digits are simply dropped.
Left shift causes binary digits to be shifted left by a specified number of digits. For example:
Left shift is equivalent to multiplication by powers of 2. As existing digits shift left, 0 digits are inserted on the right. Because integers in Python are unbounded, there are an infinite number of binary digits available, so digits on the left are never truncated or wrapped. This is a difference in behaviour compared to many other languages; we'll return to this below.
Conceptually, the bitwise complement operator ~ performs a bitwise NOT :
- bitwise complement ( conceptually ): 1 if bit is 0, else 0
In practice, ~ works this way in Python so long as you don't inspect actual bit patterns or try to compare with binary or hex literals . (That is a difference in behaviour compared to many other languages; we'll return to this difference below.) In most situations, that is not an issue.
A typical usage for bitwise operations is to manipulate bits in a flags bitfield. For example, consider the following flag definitions:
To filter a value to just these flags, you'd use bitwise and with the mask:
To check whether a given flag is set, you'd use bitwise and with the flag constant:
Note: equality comparison will be covered in lesson 5 ; if statements will be covered in lesson 6 .
To set a particular flag, you'd use bitwise or with the flag constant:
To clear the restricted flag , you'd use bitwise and together with bitwise complement:
Matching fixed bit-width assumptions of other languages
In most languages, integer data types have specific, limited bit widths, which affects how binary operations will work. But in Python, integers are not bound in bit width. This can lead to some differences in behaviour in certain situations, particularly when left shift or bitwise complement operations are involved.
For instance, in C, you could compare the result of a left shift with a literal, as in the following:
But you can't do the same directly in Python:
In C, the comparison assumes a limited bit width, with the left shift causing high-order bits to be dropped. But in Python, those bits are not dropped.
Similarly, in C, you could compare the result of a bitwise complement operation with a literal, as in the following:
But you can't do that directly in Python:
In Python, if you need to closely match bit-width assumptions in other languages, you may need to add logic that provides fixed-bit-width assumptions.
When doing a left shift operation, you can match the fixed-width assumptions of another language by applying 2**N - 1 as a mask to the result of the shift operation (where N is the assumed bit width).
When using a bitwise complement operation, you can match the fixed-width assumptions of another language by applying the result of the bitwise complement as a mask to 2**N - 1 (where N is the assumed bit width).
Representation of negative integers in Python versus other languages
When integer data types in other languages have specific, limited bit widths, negative integer values are typically represented using two's complement representation. In this representation, the value -1 has all bits set, -2 has all but the last bit set, and so on.
Signed 8-bit integers:
Because of this, the bitwise complement operation is equivalent to computing -(n + 1) , for an integer data type of a particular bit width.
For unbounded negative integers, the two's complement representation can't be assumed in practice, as it would require an infinite number of bits.
This is another form of the issue described above. We saw that results of left shift or bitwise complement operations could not be compared with literal values that involved fixed-bit-width assumptions unless accommodation to those assumptions were made. The same is true for comparing negative integers with literals that assume some fixed-width representation.
For example, the following comparison can be made in C#:
In Python, to make a similar comparison of a negative value x to a literal, add x to 2**N , where N is the assumed bit width.
Also, when the bin() function is used to convert a negative number to a binary string representation, do not expect to see the two's complement representation as you would get for similar operations in other languages. For example, compare results from C# versus Python:
The Python string has the same binary representation as +5, but with "-" prefixed.
In lesson 3, we learned that Python strings are sequences of characters. Going into more detail, strings in Python 3.x are sequences of Unicode characters. A string variable can include any Unicode character. Likewise, string literals can include any Unicode character, if supported by the console or editor you're using.
But even if they're not supported by the console or editor, any Unicode character can be written in a string literal as an escape sequence with the prefix "\u" or "\U".
The "\u" prefix is used for Unicode characters from U+0000 to U+FFFF, and exactly four hex digits must be provided: \uxxxx. The "\U" prefix can be used for any Unicode characters from U+0000 to U+10FFFF, and exactly eight hex digits must be provided: \Uxxxxxxxx.
If you learned about Unicode in the past, you might have heard about surrogate pairs : two code units in the range 0xDC00 to 0xDFFF that are combined to represent Unicode characters U+10000 and above. Forget about surrogate pairs! To specify a character from U+10000 or above in an escape sequece, you must specify the Unicode code point directly with an eight-digit \U sequence.
Python has useful built-in functions to go between a Unicode character and the integer value for its code point. The ord() function takes any single Unicode character and returns its code point as an int .
As always, numeric results in interactive mode are presented in decimal. But Unicode code points are normally cited in hex. You can use the hex() function to get a hex string representation: >>> hex(ord('€')) '0x20ac'
The chr() function is the inverse of ord() : it takes a number and returns a string with the Unicode character for that code point.
Note: The ord() function only takes a single character, not a sequence of multiple characters. Similarly, the chr() function only takes a single numeric value.
In lesson 2, we learned about the basic numeric types, int , float and complex . We also saw that numeric expressions that have a float as one of the operands always result in a float . But sometimes, you may need to get an int variable for a subsequent step. Each of the basic built-in types has a corresponding constructor function that can be used to convert between types.
The following examples show an int literal being converted to a float value using the float() constructor fuction; and float being converted to int using the int() constructor function:
Note that int() truncates the float value:
Integer and float values can be converted to complex using the complex() constructor function.
However, complex values cannot be converted to integer or float.
When working with strings, we may want to concatenate a number into a string. But Python doesn't allow an int , float or complex to be concatenated onto a string.
But we can use the built-in str() constructor function to convert numbers into strings. This works for any of the numeric types:
Note: Integrating numbers into strings, or string formatting , is a bigger topic, and there are other ways to do that with more and better options. We'll save that for a later lesson.
We can use int() and float() to convert strings to numbers, so long as the strings are string expressions of decimal numbers:
Whitespace within the string before or after the number is tolerated, but there must be only one number and no non-numeric characters:
Earlier, we saw that integer literals can be expressed in hex, binary or octal as well as in decimal. When using int() to convert from a string, however, the number string can only be in decimal form.
The "_" grouping delimiter can be included in the string:
We've already seen that strings in Python 3.x use Unicode. Unicode supports many scripts that have their own decimal digit characters. In Python, conversion from number strings is supported for any characters that Unicode defines as decimal digits. In the following example, a number string using Arabic-script digits is converted to an int :
You can even mix and match digits from different scripts—though this isn't recommended:
There's more we could cover on numbers and strings, but it's time for something else. In the next lesson , we'll learn about another booleans and logical expressions.
CopyAssignment
We are Python language experts, a community to solve Python problems, we are a 1.2 Million community on Instagram, now here to help with our blogs.
- Shift Numbers in Python | Assignment Expert
Hello friends, in this article you will learn how to shift numbers in Python in a string from any place of string to the start of the string. For example, making bnj67jbn to 67bnjjbn , simply we need to remove integers from inside of a string and place them to start of the string. To check whether a character is a digit or not, we can use isdigit() method of Python.
Code to shift numbers in Python:

Explanation:
So, we created a string, declared two empty lists, then inside for loop, we checked each character of string whether it is digit or not. If its a digit, we will append it to list 1 else list 2. At last, we are printing both lists by converting them to strings(using join() method) and joining them with + operator.
- Hyphenate Letters in Python
- Earthquake in Python | Easy Calculation
- Striped Rectangle in Python
- Perpendicular Words in Python
- Free shipping in Python
- Raj has ordered two electronic items Python | Assignment Expert
- Team Points in Python
- Ticket selling in Cricket Stadium using Python | Assignment Expert
- Split the sentence in Python
- String Slicing in JavaScript
- First and Last Digits in Python | Assignment Expert
- List Indexing in Python
- Date Format in Python | Assignment Expert
- New Year Countdown in Python
- Add Two Polynomials in Python
- Sum of even numbers in Python | Assignment Expert
- Evens and Odds in Python
- A Game of Letters in Python
- Sum of non-primes in Python
- Smallest Missing Number in Python
- String Rotation in Python
- Secret Message in Python
- Word Mix in Python
- Single Digit Number in Python
- Weekend in Python
- Temperature Conversion in Python
- Special Characters in Python
- Sum of Prime Numbers in the Input in Python

Author: Harry
Search…..
Machine Learning
Data Structures and Algorithms(Python)
Python Turtle
Games with Python
All Blogs On-Site
Python Compiler(Interpreter)
Online Java Editor
Online C++ Editor
Online C Editor
All Editors
Services(Freelancing)
Recent Posts
- Imagination to Reality, Unlocking the Future: Genesis Physics Engine for 4D Simulation
- Simple Code to compare Speed of Python, Java, and C++?
- Falling Stars Animation on Python.Hub October 2024
- Most Underrated Database Trick | Life-Saving SQL Command
- Python List Methods
© Copyright 2019-2024 www.copyassignment.com. All rights reserved. Developed by copyassignment
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Python | Extract Numbers from String
Many times, while working with strings we come across this issue in which we need to get all the numeric occurrences. This type of problem generally occurs in competitive programming and also in web development. Let’s discuss certain ways in which this problem can be solved in Python .
Use a loop to iterate over each character in the string and check if it is a digit using the isdigit() method. If it is a digit, append it to a list.
Table of Content
Extract numbers from string using List Comprehension
Extract interger from string using isnumeric() method, extract digit from string using regex, extract digit from string using filter() function, extract numbers from string using str.translate() with str.maketrans() .
This problem can be solved by using the split function to convert string to list and then the list comprehension which can help us iterate through the list and isdigit function helps to get the digit out of a string.
In Python, we have isnumeric function which can tell the user whether a particular element is a number or not so by this method we can also extract the number from a string.
This particular problem can also be solved using Python regex, we can use the findall function to check for the numeric occurrences using a matching regex string.
First, we define the input string then print the original string and split the input string into a list of words using the split() method. Use the filter() function to filter out non-numeric elements from the list by applying the lambda function x .isdigit() to each elementConvert the remaining elements in the filtered list to integers using a list comprehension. Print the resulting list of integers
Define the input string then Initialize a translation table to remove non-numeric characters using str. maketrans() . Use str. translate() with the translation table to remove non-numeric characters from the string and store the result in a new string called numeric_string . Use str. split() to split the numeric_string into a list of words and store the result in a new list called words. Initialize an empty list called numbers to store the resulting integers and then iterate over each word in the list of words. Check if the word is a numeric string using str. isdigit() .If the word is a numeric string, convert it to an integer using int() and append it to the list of numbers.
Similar Reads
- Python | Extract Numbers from String Many times, while working with strings we come across this issue in which we need to get all the numeric occurrences. This type of problem generally occurs in competitive programming and also in web development. Let's discuss certain ways in which this problem can be solved in Python. Example Use a 5 min read
- Python | Extract numbers from list of strings Sometimes, we can data in many forms and we desire to perform both conversions and extractions of certain specific parts of a whole. One such issue can be extracting a number from a string and extending this, sometimes it can be more than just an element string but a list of it. Let's discuss certai 8 min read
- Python - Extract Percentages from String Given a String, extract all the numbers that are percentages. Input : test_str = 'geeksforgeeks 20% is 100% way to get 200% success' Output : ['20%', '100%', '200%'] Explanation : 20%, 100% and 200% are percentages present. Input : test_str = 'geeksforgeeks is way to get success' Output : [] Explana 2 min read
- Python | Extract digits from given string While programming, sometimes, we just require a certain type of data and need to discard other. This type of problem is quite common in Data Science domain, and since Data Science uses Python worldwide, its important to know how to extract specific elements. This article discusses certain ways in wh 3 min read
- Python | Extract Numbers in Brackets in String Sometimes, while working with Python strings, we can have a problem in which we have to perform the task of extracting numbers in strings that are enclosed in brackets. Let's discuss the certain ways in which this task can be performed. Method 1: Using regex The way to solve this task is to construc 6 min read
- Python Check If String is Number In Python, there are different situations where we need to determine whether a given string is valid or not. Given a string, the task is to develop a Python program to check whether the string represents a valid number. Example: Using isdigit() Method [GFGTABS] Python # Python code to check if strin 6 min read
- Python | Insert a number in string Sometimes, while dealing with strings, we may encounter a problem in which we might have a numeric variable whose value keeps changing and we need to print the string including that number. Strings and numbers being different data types have to be solved in different ways. Let's discuss specific way 4 min read
- Python - Extract date in String Given a string, the task is to write a Python program to extract date from it. Input : test_str = "gfg at 2021-01-04" Output : 2021-01-04 Explanation : Date format string found. Input : test_str = "2021-01-04 for gfg" Output : 2021-01-04 Explanation : Date format string found. Method #1 : Using re.s 4 min read
- Python | Frequency of numbers in String Sometimes, while working with Strings, we can have a problem in which we need to check how many of numerics are present in strings. This is a common problem and have application across many domains like day-day programming and data science. Lets discuss certain ways in which this task can be perform 4 min read
- Convert Complex Number to String in Python We are given complex numbers and we have to convert these complex numbers into strings and return the result in string form. In this article, we will see how we can convert complex numbers to strings in Python. Examples: Input : 3+4j <complex>Output : 3+4j <string> Explanation: We can se 3 min read
- Python - Extract range characters from String Given a String, extract characters only which lie between given letters. Input : test_str = 'geekforgeeks is best', strt, end = "g", "s" Output : gkorgksiss Explanation : All characters after g and before s are retained. Input : test_str = 'geekforgeeks is best', strt, end = "g", "r" Output : gkorgk 4 min read
- Python - Retain Numbers in String Sometimes, while working with Python Strings, we can have a problem in which we need to perform the removal of all the characters other than integers. This kind of problem can have application in many data domains such as Machine Learning and web development. Let's discuss certain ways in which this 11 min read
- Python - Extract Tuples with all Numeric Strings Given a list of tuples, extract only those tuples which have all numeric strings. Input : test_list = [("45", "86"), ("Gfg", "1"), ("98", "10")] Output : [('45', '86'), ('98', '10')] Explanation : Only number representing tuples are filtered. Input : test_list = [("Gfg", "1")] Output : [] Explanatio 5 min read
- Extract Substrings From A List Into A List In Python Python is renowned for its simplicity and versatility, making it a popular choice for various programming tasks. When working with lists, one common requirement is to extract substrings from the elements of the list and organize them into a new list. In this article, we will see how we can extract s 2 min read
- Python | Get positional characters from String Sometimes, while working with Python strings, we can have a problem in which we need to create a substring by joining the particular index elements from a string. Let's discuss certain ways in which this task can be performed. Method #1: Using loop This is a brute method in which this task can be pe 5 min read
- Extract Multiple JSON Objects from one File using Python Python is extremely useful for working with JSON( JavaScript Object Notation) data, which is a most used format for storing and exchanging information. However, it can become challenging when dealing with multiple JSON objects stored within a single file. In this article, we will see some techniques 3 min read
- Python | Lowercase first character of String The problem of capitalizing a string is quite common and has been discussed many times. But sometimes, we might have a problem like this in which we need to convert the first character of the string to lowercase. Let us discuss certain ways in which this can be performed. Method #1: Using string sli 4 min read
- Extract all integers from a given String Given a string str, extract all integers words from it. Example: Input: str = "1Hello2 &* how are y5ou"Output: 1 2 5 Input: str = "Hey everyone, I have 500 rupees and I would spend a 100"Output: 500 100 Approach: To solve this problem follow the below steps: Create a string tillNow, which will s 9 min read
- Python - Extract digits from Tuple list Sometimes, while working with Python lists, we can have a problem in which we need to perform extraction of all the digits from tuple list. This kind of problem can find its application in data domains and day-day programming. Let's discuss certain ways in which this task can be performed. Input : t 6 min read
- Python Programs
- Python string-programs
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- How it works
- Homework answers
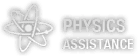
Answer to Question #188507 in Python for mani
Numbers in String - 2
Given a string, write a program to return the sum and average of the numbers that appear in the string, ignoring all other characters.
The input will be a single line containing a string.
The output should contain the sum and average of the numbers that appear in the string.
Note: Round the average value to two decimal places.
Explanation
For example, if the given string is "I am 25 years and 10 months old", the numbers are 25, 10. Your code should print the sum of the numbers(35) and the average of the numbers(17.5) in the new line.
Sample Input 1
I am 25 years and 10 months old
Sample Output 1
Sample Input 2
Tech Foundation 35567
Sample Output 2
Need a fast expert's response?
and get a quick answer at the best price
for any assignment or question with DETAILED EXPLANATIONS !
Leave a comment
Ask your question, related questions.
- 1. Add two polynomialsGiven two polynomials A and B, write a program that adds the given two polynomial
- 2. Area of Largest Rectangle in HistogramGiven an list of integer heights representing the histogram
- 3. Using while loop and If statements, print all the letters in the following string except for the let
- 4. Given a string, write a program to print a secret message that replaces characters with numbers
- 5. Using while loop and If statements, print all the letters in the following string except for the let
- 6. Write a program that takes an input letter from the user until a vowel is entered. Use infinite lo
- 7. Write a program that rolls a dice until the user chooses to exit the program. Use random module to g
- Programming
- Engineering
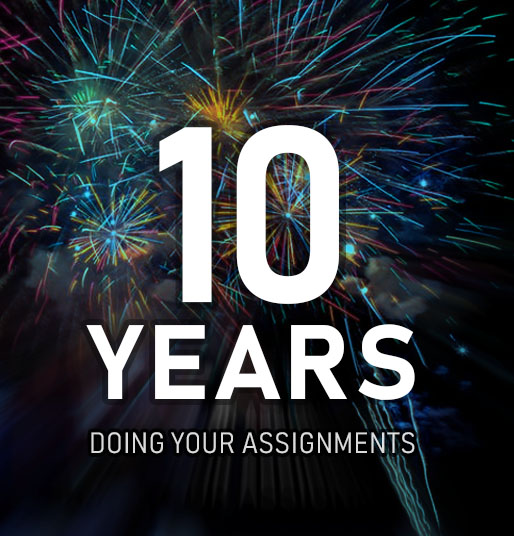
Who Can Help Me with My Assignment
There are three certainties in this world: Death, Taxes and Homework Assignments. No matter where you study, and no matter…
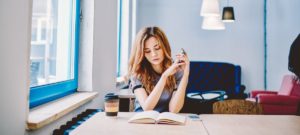
How to Finish Assignments When You Can’t
Crunch time is coming, deadlines need to be met, essays need to be submitted, and tests should be studied for.…
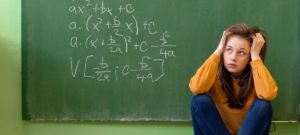
How to Effectively Study for a Math Test
Numbers and figures are an essential part of our world, necessary for almost everything we do every day. As important…

IMAGES
COMMENTS
For example, if the given string is "I am 25 years and 10 months old", the numbers are 25, 10. Your code should print the sum of the numbers(35) and the average of the numbers(17.5) in the new line. Sample Input 1. I am 25 years and 10 months old. Sample Output 1. 35. 17.5. Sample Input 2. Tech Foundation 35567. Sample Output 2. 35567. 35567.0
Mar 12, 2021 · The output should contain the sum and average of the numbers that appear in the string. Note: Round the average value to two decimal places.Explanation. For example, if the given string is "I am 25 years and 10 months old", the numbers are 25, 10. Your code should print the sum of the numbers(35) and the average of the numbers(17.5) in the new ...
Mar 24, 2021 · The output should contain the sum and average of the numbers that appear in the string. Note: Round the average value to two decimal places.Explanation. For example, if the given string is "I am 25 years and 10 months old", the numbers are 25, 10. Your code should print the sum of the numbers(35) and the average of the numbers(17.5) in the new ...
We've already seen that strings in Python 3.x use Unicode. Unicode supports many scripts that have their own decimal digit characters. In Python, conversion from number strings is supported for any characters that Unicode defines as decimal digits. In the following example, a number string using Arabic-script digits is converted to an int:
Feb 28, 2024 · How To Numbers In String-2 In Python Assignment Expert Like An Expert/ Pro! It was my first time doing numerical arithmetic in python, and not only I can visualize data with graphs and formulas, but I also understand the logical workings in case you think of Python like JMP.
Aug 26, 2022 · Hello friends, in this article you will learn how to shift numbers in Python in a string from any place of string to the start of the string. For example, making bnj67jbn to 67bnjjbn, simply we need to remove integers from inside of a string and place them to start of the string.
Sep 16, 2024 · Extract numbers from string using List Comprehension. This problem can be solved by using the split function to convert string to list and then the list comprehension which can help us iterate through the list and isdigit function helps to get the digit out of a string. Python
Python Problem: Number in string 1 | Grand Assignment 4 | Python Coding & Debugging | Nxtwave CCBP 4.0 | Coding Tips & TricksTitle: "Sum and Average of Numb...
May 3, 2021 · Numbers in String - 2. Given a string, write a program to return the sum and average of the numbers that appear in the string, ignoring all other characters. Input. The input will be a single line containing a string. Output. The output should contain the sum and average of the numbers that appear in the string.
Jan 31, 2017 · I need to extract two numbers inside a string. They can look like this: (0,0) (122,158) (1,22) (883,8) etc... So I want to get the first number before the comma and the number after the comma and store them in variables. I can get the first number like this: myString.split(',')[0][1:]) However, I can't figure out how to get the next number.